Encrypt Connection String in Web.Config
Encrypt App Settings in Web.Config
In .Net application development we use Web.Config file to store sensitive information like Database Connection, Email, and SMTP etc. But Web.Config is readable and can be easily read by anyone.
Thinks about a scenario when someone got Web.Config file. He can easily access your database and etc. because all information available on Web.Config file.
So it’s better to encrypt the connection string in Web.Config to increase the security.
Today we are going to show how to encrypt connection string in Web.Config file.
We have two ways to encrypt connection string
- Use ASP.NET IIS Registration Tool (Aspnet_regiis.exe) to encrypt connection string
- Use ConnectionStringsSection class
This is our web.config file
Use Aspnet_regiis.exe to encrypt connection string - Steps to encrypt connection string with the help of Aspnet_regiis.exe tools.
We have options to encrypt web.config physical path or Web.config when application hosted on IIS.
- Encrypt Web.config in physical Path
- Encrypt Web.config when application hosted on IIS
Encrypt Web.config in physical Path
1. Open Command Prompt with Administrator privileges
2. Run following command into Command Prompt
aspnet_regiis -pef "connectionStrings" D:\EncryptConnectionString\EncryptConnectionString"
aspnet_regiis take three parameters
1. –pef - Encrypts the specified configuration section of the Web.config file in the specified physical (not virtual) directory.
2. “connectionString” – Its section to encrypt its case sensitive.
3. Physical Path of application - Define application physical path. If your application located in “D:\EncryptConnectionString\EncryptConnectionString” directory then use “D:\EncryptConnectionString\EncryptConnectionString”.
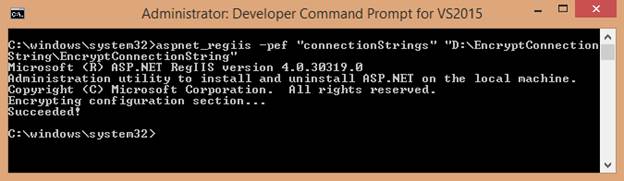
Encrypt Web.config when application hosted on IIS
1. Open Command Prompt with Administrator privileges
2. Run following command into Command Prompt
aspnet_regiis -pe "connectionStrings" -app "/EncryptConnectionString" -prov "RsaProtectedConfigurationProvider"
Note: You can learn more about options in aspnet_regiis here - https://msdn.microsoft.com/en-us/library/k6h9cz8h.aspx
• Use ConnectionStringsSection class – If you don’t want to use aspnet_regiis tools and want to encrypt you Web.Config file when application start. We can write code in Global.asax on Application_Start() event to encrypt Web.Config .
We need to follow following steps
- Use WebConfigurationManager to get Configuration
- Get all connection string list by GetSection Method
- Use ProtectSection method to Encrypt Connection string
- Save Web.config file
1. Use WebConfigurationManager to get Configuration
Configuration configuration = WebConfigurationManager.OpenWebConfiguration("~");
2. Get all connection string list by GetSection Method
ConnectionStringsSection connectionStringsSection = (ConnectionStringsSection)configuration.GetSection("connectionStrings");
3. Use ProtectSection method to Encrypt Connection string
ProtectSection("DataProtectionConfigurationProvider");
4. Save Web.config file - SectionInformation.ForceSave = true;
Example
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Linq;
using System.Web;
using System.Web.Configuration;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
namespace Encrypt_Connection_String
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
Configuration configuration = WebConfigurationManager.OpenWebConfiguration("~");
ConnectionStringsSection connectionStringsSection = (ConnectionStringsSection)configuration.GetSection("connectionStrings");
AppSettingsSection appSettingsSection = (AppSettingsSection)configuration.GetSection("appSettings");
EncryptConnectionStrings(configuration, connectionStringsSection);
EncryptAppSettings(configuration, appSettingsSection);
}
//Encrypt Connection string
private static void EncryptConnectionStrings(Configuration config, ConnectionStringsSection connectionStringsSection)
{
if (!connectionStringsSection.SectionInformation.IsProtected)
{
connectionStringsSection.SectionInformation.ProtectSection("DataProtectionConfigurationProvider");
connectionStringsSection.SectionInformation.ForceSave = true;
}
config.Save(ConfigurationSaveMode.Modified);
}
//Encrypt App Settings
private static void EncryptAppSettings(Configuration config, AppSettingsSection appSettingsSection)
{
if (!appSettingsSection.SectionInformation.IsProtected)
{
appSettingsSection.SectionInformation.ProtectSection("DataProtectionConfigurationProvider");
appSettingsSection.SectionInformation.ForceSave = true;
}
config.Save(ConfigurationSaveMode.Modified);
}
}
}
Keywords
Comments
Post a Comment