When we are using AngularJs for client side development. We are not able to call any $scope function directly from console like we can call any function in Java script and in jQuery.
Following we have one example of simple AngularJs Page. Having one Java Script, One JQuery and Two AngularJs $scope function
Java Script Function – JsTest()
JQuery Function – JQueryTest()
AngularJs Function -
$scope.showHello()
$scope.sum()
Now we will call all function from console for this open following program in Google Chrome. And right click anywhere in web page and select “Inspect element”.
It will show you screen like this.
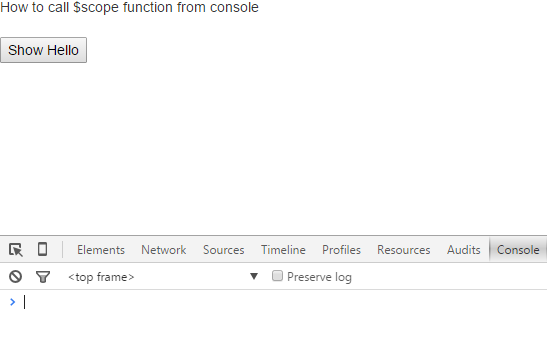
Example
index.html
<!DOCTYPE html>
<html>
<head>
<title>How to call $scope function from console</title>
<link rel="stylesheet" href="http://getbootstrap.com/2.3.2/assets/css/bootstrap.css">
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.4/angular.js"></script>
<script src="//code.jquery.com/jquery-1.11.3.min.js"></script>
<script>
var app = angular.module("myApp", []);
app.controller("MyDemoController", function($scope) {
$scope.message = "How to call $scope function from console";
$scope.showHello = function() {
alert("Hello Angular");
}
$scope.sum = function(value1, value2) {
return value1 + value2;
}
});
function JsTest() {
alert("Js Hello");
}
$(document).ready(function() {
$.fn.JQueryTest = function() {
alert("JQuery Hello");
};
});
</script>
</head>
<body>
<div ng-app="myApp" ng-controller="MyDemoController" id="myDiv">
<div id="msg">
{{message}}
<br />
</div>
<br>
<button ng-click="showHello()">Show Hello</button>
</div>
</body>
</html>
Now go into “console” and follow following steps
Call Java Script function from console
For call Java Script function from console we just need write “JsTest()” into console. Like thisOutput:
Call JQuery function from console
For call JQuery Function from console use JQuery function like this “$().JQueryTest()”. Like thisOutput:
Call AngularJs $scope function from Console
Now call two AngularJs $Scope function from console. For call AngularJs functions use following command into console:Syntax
angular.element(document.querySelector('#someID')).scope().someFunction();
#someID – Can be any element’s ID which exists inside ng-controller. But it’s better to use id of element where use using ng-controller. Like we are using myDiv’s ID.
angular.element(document.querySelector('#myDiv')).scope().showHello();
It will show you “Alert Box” having message “Hello AngularJs” Like this
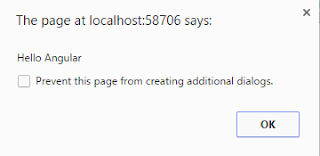
We have another $scope function called “Sum”, Now we will call this using following command
angular.element(document.querySelector('#myDiv')).scope().sum(100,100);
It will return you “200” into console. Like this
You also have option to assign output to variable like we assign output into Sum variable .
Keywords –
Comments
Post a Comment