How to show and customize popup in ionic framework
Ionic framework allow us to show popup or dialog box programmatically. popup is use to take required input from user and take desired action.
For example - User deleting a record , Application show a popup ( confirm box ) to insure that user really want to delete a record Or user clicked delete button by mistake.
For open a popup you should inject "$ionicPopup" dependency into your controller.
Ionic Show - Show function is main function for all pupups.
Ionic framework provide three types of popup
- Alert
- Prompt
- Confirm
Ionic Alert - Alert is used for show a simple alert popup with a title ,message and a button . Use can click to close the popup.
Alert mostly used to show warning. And default "okText" is "OK".
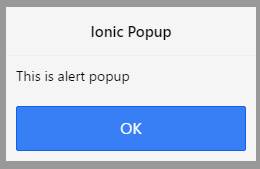
01
02
03
04
05
06
07
08
09
10
| // Alert popup code $scope.showAlert = function () { var alertPopup = $ionicPopup.alert({ title: 'Ionic Popup' , template: 'This is alert popup' , }); alertPopup.then( function (res) { console.log( 'Thanks' ); }); }; |
// Alert popup code $scope.showAlert = function() { var alertPopup = $ionicPopup.alert({ title: 'Ionic Popup', template: 'This is alert popup', }); alertPopup.then(function(res) { console.log('Thanks'); }); };
We can customize ionic alert popup to set following options.
1
2
3
4
5
6
7
8
9
| { title: '' , // String. The title of the popup. cssClass: '' , // String, The custom CSS class name subTitle: '' , // String (optional). The sub-title of the popup. template: '' , // String (optional). The html template to place in the popup body. templateUrl: '' , // String (optional). The URL of an html template to place in the popup body. okText: '' , // String (default: 'OK'). The text of the OK button. okType: '' , // String (default: 'button-positive'). The type of the OK button. } |
{ title: '', // String. The title of the popup. cssClass: '', // String, The custom CSS class name subTitle: '', // String (optional). The sub-title of the popup. template: '', // String (optional). The html template to place in the popup body. templateUrl: '', // String (optional). The URL of an html template to place in the popup body. okText: '', // String (default: 'OK'). The text of the OK button. okType: '', // String (default: 'button-positive'). The type of the OK button. }
Ionic Confirm - Ionic confirm show a simple popup with "Cancel" and "Ok" button. We can get user selection on promise true if the user presses the OK button, and false if the user presses the Cancel button.
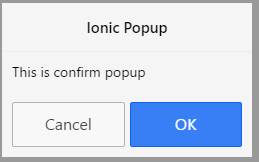
01
02
03
04
05
06
07
08
09
10
11
12
13
14
| // Confirm popup code $scope.showConfirm = function () { var confirmPopup = $ionicPopup.confirm({ title: 'Ionic Popup' , template: 'This is confirm popup' , }); confirmPopup.then( function (res) { if (res) { console.log( 'You clicked on "OK" button' ); } else { console.log( 'You clicked on "Cancel" button' ); } }); }; |
// Confirm popup code $scope.showConfirm = function() { var confirmPopup = $ionicPopup.confirm({ title: 'Ionic Popup', template: 'This is confirm popup', }); confirmPopup.then(function(res) { if (res) { console.log('You clicked on "OK" button'); } else { console.log('You clicked on "Cancel" button'); } }); };
We can customize ionic confirm popup to set following options.
01
02
03
04
05
06
07
08
09
10
11
| { title: '' , // String. The title of the popup. cssClass: '' , // String, The custom CSS class name subTitle: '' , // String (optional). The sub-title of the popup. template: '' , // String (optional). The html template to place in the popup body. templateUrl: '' , // String (optional). The URL of an html template to place in the popup body. cancelText: '' , // String (default: 'Cancel'). The text of the Cancel button. cancelType: '' , // String (default: 'button-default'). The type of the Cancel button. okText: '' , // String (default: 'OK'). The text of the OK button. okType: '' , // String (default: 'button-positive'). The type of the OK button. } |
{ title: '', // String. The title of the popup. cssClass: '', // String, The custom CSS class name subTitle: '', // String (optional). The sub-title of the popup. template: '', // String (optional). The html template to place in the popup body. templateUrl: '', // String (optional). The URL of an html template to place in the popup body. cancelText: '', // String (default: 'Cancel'). The text of the Cancel button. cancelType: '', // String (default: 'button-default'). The type of the Cancel button. okText: '', // String (default: 'OK'). The text of the OK button. okType: '', // String (default: 'button-positive'). The type of the OK button. }
Prompt - Ionic prompt show a simple prompt popup, with input, OK button, and Cancel button. We can get user action on promise when user insert any value into text box and click OK button. But if user presses Cancel button then promise return undefined.
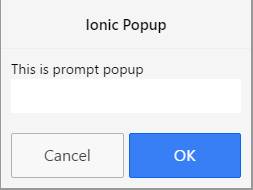
01
02
03
04
05
06
07
08
09
10
11
12
13
14
| // Prompt popup code $scope.showPrompt = function () { var promptPopup = $ionicPopup.prompt({ title: 'Ionic Popup' , template: 'This is prompt popup' }); promptPopup.then( function (res) { if (res) { console.log( 'Your input is ' , res); } else { console.log( 'Please enter input' ); } }); }; |
// Prompt popup code $scope.showPrompt = function() { var promptPopup = $ionicPopup.prompt({ title: 'Ionic Popup', template: 'This is prompt popup' }); promptPopup.then(function(res) { if (res) { console.log('Your input is ', res); } else { console.log('Please enter input'); } }); };
We can customize ionic prompt popup to set following options.
01
02
03
04
05
06
07
08
09
10
11
12
13
| { title: '' , // String. The title of the popup. cssClass: '' , // String, The custom CSS class name subTitle: '' , // String (optional). The sub-title of the popup. template: '' , // String (optional). The html template to place in the popup body. templateUrl: '' , // String (optional). The URL of an html template to place in the popup body. inputType: // String (default: 'text'). The type of input to use inputPlaceholder: // String (default: ''). A placeholder to use for the input. cancelText: // String (default: 'Cancel'. The text of the Cancel button. cancelType: // String (default: 'button-default'). The type of the Cancel button. okText: // String (default: 'OK'). The text of the OK button. okType: // String (default: 'button-positive'). The type of the OK button. } |
{ title: '', // String. The title of the popup. cssClass: '', // String, The custom CSS class name subTitle: '', // String (optional). The sub-title of the popup. template: '', // String (optional). The html template to place in the popup body. templateUrl: '', // String (optional). The URL of an html template to place in the popup body. inputType: // String (default: 'text'). The type of input to use inputPlaceholder: // String (default: ''). A placeholder to use for the input. cancelText: // String (default: 'Cancel'. The text of the Cancel button. cancelType: // String (default: 'button-default'). The type of the Cancel button. okText: // String (default: 'OK'). The text of the OK button. okType: // String (default: 'button-positive'). The type of the OK button. }
Ionic Show - Ionic show is used to show more complex popup. As we know that its main function for all popups.
Show method very useful when we need bind ionic popups with our model.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
| // showpopup method code $scope.showPopup = function () { $scope.data = {} var myPopup = $ionicPopup.show({ template: ' Enter Password<input type="password" ng-model="data.userPassword"> <br> Enter Confirm Password <input type="password" ng-model="data.confirmPassword" > ' , title: 'Enter Password' , subTitle: 'Please use normal things' , scope: $scope, buttons: [{ text: 'Cancel' }, { text: '<b>Save</b>' , type: 'button-positive' , onTap: function (e) { if (!$scope.data.userPassword) { //don't allow the user to close unless he enters wifi password e.preventDefault(); } else { return $scope.data; } } }, ] }); myPopup.then( function (res) { if (res) { if (res.userPassword == res.confirmPassword) { console.log('Password Is Ok '); } else { console.log(' Password not matched '); } } else { console.log(' Enter password'); } }); }; |
// showpopup method code $scope.showPopup = function() { $scope.data = {} var myPopup = $ionicPopup.show({ template: ' Enter Password<input type="password" ng-model="data.userPassword"> <br> Enter Confirm Password <input type="password" ng-model="data.confirmPassword" > ', title: 'Enter Password', subTitle: 'Please use normal things', scope: $scope, buttons: [{ text: 'Cancel' }, { text: '<b>Save</b>', type: 'button-positive', onTap: function(e) { if (!$scope.data.userPassword) { //don't allow the user to close unless he enters wifi password e.preventDefault(); } else { return $scope.data; } } }, ] }); myPopup.then(function(res) { if (res) { if (res.userPassword == res.confirmPassword) { console.log('Password Is Ok'); } else { console.log('Password not matched'); } } else { console.log('Enter password'); } }); };
We can customize ionic show method to set following options.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| { title: '' , // String. The title of the popup. cssClass: '' , // String, The custom CSS class name subTitle: '' , // String (optional). The sub-title of the popup. template: '' , // String (optional). The html template to place in the popup body. templateUrl: '' , // String (optional). The URL of an html template to place in the popup body. scope: null , // Scope (optional). A scope to link to the popup content. buttons: [{ // Array[Object] (optional). Buttons to place in the popup footer. text: 'Cancel' , type: 'button-default' , onTap: function (e) { // e.preventDefault() will stop the popup from closing when tapped. e.preventDefault(); } }, { text: 'OK' , type: 'button-positive' , onTap: function (e) { // Returning a value will cause the promise to resolve with the given value. return scope.data.response; } }] } |
{ title: '', // String. The title of the popup. cssClass: '', // String, The custom CSS class name subTitle: '', // String (optional). The sub-title of the popup. template: '', // String (optional). The html template to place in the popup body. templateUrl: '', // String (optional). The URL of an html template to place in the popup body. scope: null, // Scope (optional). A scope to link to the popup content. buttons: [{ // Array[Object] (optional). Buttons to place in the popup footer. text: 'Cancel', type: 'button-default', onTap: function(e) { // e.preventDefault() will stop the popup from closing when tapped. e.preventDefault(); } }, { text: 'OK', type: 'button-positive', onTap: function(e) { // Returning a value will cause the promise to resolve with the given value. return scope.data.response; } }] }
Awesome post about Ionic, thank you, very helpful!
ReplyDeleteHey,
ReplyDeleteIs possible to copy text from ionic popup?